Efficiently Create and Fill Pandas DataFrames in Python
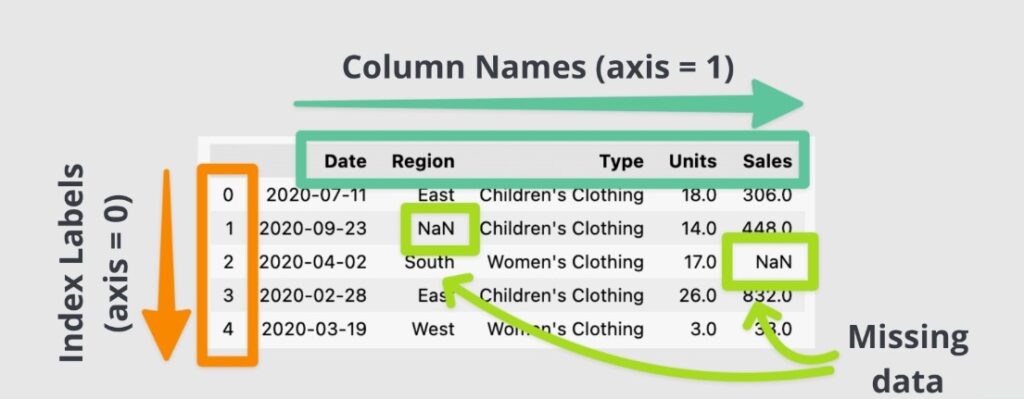
Efficiently create and fill Pandas DataFrames, pandas is a popular Python library for data manipulation and analysis, widely used by data scientists and analysts. Often, beginners and even experienced developers face challenges when working with Pandas DataFrames, particularly when it comes to efficiently creating and filling them. Questions like “How to build and fill a Pandas DataFrame from a for loop?”, “What’s the best way to add rows to a DataFrame?”, and “How do I create an empty Pandas DataFrame?” . In this blog, we’ll explore efficient ways to create and populate Pandas DataFrames. We’ll provide practical solutions, including code examples for creating empty DataFrames, appending rows, and filling DataFrames using loops.
Why Efficiency Matters When Handling DataFrames
When searching for efficiently create and fill Pandas DataFrames, you can consider using techniques like appending dictionaries or lists. Creating and filling DataFrames efficiently is crucial for optimizing performance when working with large datasets. Using inefficient methods can significantly slow down your script, especially when dealing with millions of rows. By adopting the right techniques, you can reduce execution time, improve memory usage, and streamline your data processing workflows.
Efficient Ways to Add Rows to a Pandas DataFrame
1. Creating an Empty Pandas DataFrame
The first step to working with DataFrames is often creating an empty one. You can initialize a DataFrame with no rows and predefined column names. Here’s an example:
import pandas as pd
# Create an empty DataFrame with specified column names
df = pd.DataFrame(columns=['Name', 'Age', 'City'])
print(df)
# Output:
# Empty DataFrame
# Columns: [Name, Age, City]
# Index: []
This approach is useful for scenarios where you know the structure of your data but need to populate it later.
2. Appending Rows to a DataFrame
One common method is appending rows to a DataFrame within a loop. However, the naive approach using pd.DataFrame.append()
is inefficient for large datasets. Here’s an example of the inefficient method:
# Inefficient method: Using .append() in a loop
for i in range(5):
df = df.append({'Name': f'Person{i}', 'Age': 20 + i, 'City': f'City{i}'}, ignore_index=True)
print(df)
# Output:
# Name Age City
# 0 Person0 20 City0
# 1 Person1 21 City1
# 2 Person2 22 City2
# 3 Person3 23 City3
# 4 Person4 24 City4
While this works for small datasets, it can be extremely slow for larger ones due to the overhead of creating a new DataFrame every time you append.
The Efficient Alternative: Building a List of Dictionaries
To efficiently add rows, build a list of dictionaries and then create a DataFrame in one step. This avoids repeated memory allocations.
# Efficient method: Using a list of dictionaries
data = []
# Build the list
for i in range(5):
data.append({'Name': f'Person{i}', 'Age': 20 + i, 'City': f'City{i}'})
# Create DataFrame in one step
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age City
# 0 Person0 20 City0
# 1 Person1 21 City1
# 2 Person2 22 City2
# 3 Person3 23 City3
# 4 Person4 24 City4
This method is much faster and more memory-efficient.
Building and Filling a DataFrame Using Loops
Sometimes, your data comes from a dynamic source, and you need to process it in a loop. Below is an example of creating and filling a DataFrame dynamically:
# Example: Filling a DataFrame dynamically in a loop
columns = ['Product', 'Price', 'Quantity']
df = pd.DataFrame(columns=columns)
data = [
('Laptop', 1000, 5),
('Phone', 800, 10),
('Tablet', 400, 15)
]
# Add rows dynamically
for item in data:
df = pd.concat([df, pd.DataFrame([item], columns=columns)], ignore_index=True)
print(df)
# Output:
# Product Price Quantity
# 0 Laptop 1000 5
# 1 Phone 800 10
# 2 Tablet 400 15
This approach uses pd.concat()
for better performance compared to append()
.
Using NumPy Arrays for Performance
If you’re working with numeric data, consider using NumPy arrays to create and populate your DataFrame. NumPy arrays are faster for bulk operations, and you can easily convert them to DataFrames.
import numpy as np
# Create a NumPy array
data = np.array([
['Alice', 25, 'New York'],
['Bob', 30, 'Chicago'],
['Charlie', 35, 'Los Angeles']
])
# Convert to a DataFrame
df = pd.DataFrame(data, columns=['Name', 'Age', 'City'])
print(df)
# Output:
# Name Age City
# 0 Alice 25 New York
# 1 Bob 30 Chicago
# 2 Charlie 35 Los Angeles
This method is particularly useful for numerical and structured datasets.
Key Takeaways
- Avoid Using
append
in Loops: Instead, build a list of dictionaries or usepd.concat()
for better performance. - Predefine DataFrame Structure: Always define column names upfront to maintain clarity and avoid errors.
- Leverage NumPy for Speed: Use NumPy arrays when working with large numeric datasets for better efficiency.